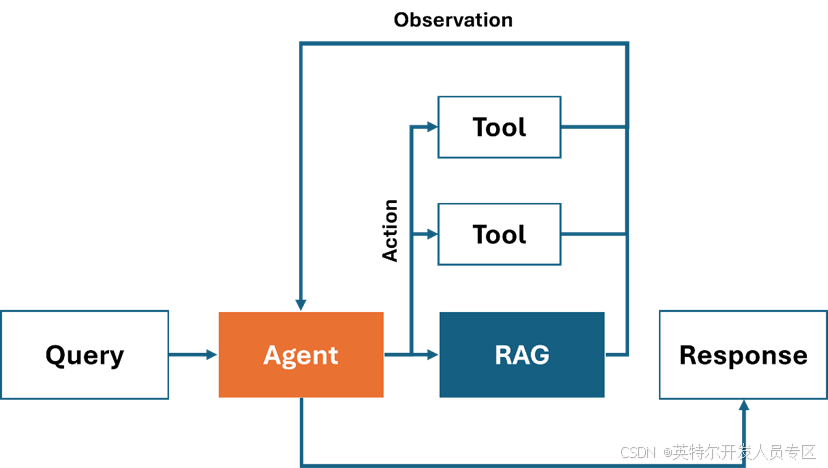
使用OpenVINO和LlamaIndex构建Agentic-RAG系统
通过将Agent和RAG进行结合,我们直接提升LLM在解决复杂任务时的能力,相较于传统的RAG,Agentic-RAG更具产业落地价值。同时随着多智能体方法的引入,基于Agent的RAG将逐步取代传统RAG系统,实现更灵活,更精确的大语言模型应用业务体系。
杨亦诚
背景
RAG系统的全称是Retrieval-augmented Generation,本质上是Prompt Engineering,通过在Prompt中注入检索得到的外部数据,可以有效地解决大语言模型在知识时效性和专业性上的不足。但同时传统的RAG系统也有它的缺陷,例如灵活性较差,由于RAG会过分依赖于向量数据库的检索结果,导致其在解决一些复杂问题的时候,只是一味地 “搬运” 检索结果,无法通过推理找到更优的解决途径,此外随着向量数据库的规模增大,传统RAG也无法高效对输入请求进行分类和过滤,导致检索过程犹如 “大海捞针” ,费时费力。
图:Agentic-RAG系统示例
而基于AI智能体的RAG系统(以下简称Agentic-RAG)恰好可以解决传统RAG在灵活性上的不足,它通过将多个不同类别的RAG检测器,以工具的形式集成在AI智能体中,让AI智能体根据用户的请求,判断是否需要调用RAG搜索上下文,以及调用哪个RAG工具进行检索,例如在回答一个历史相关的问题时,Agentic-RAG就会优先在历史类的RAG检索器中搜索答案,又或是在回答一个涉及数学计算的问题时,Agentic-RAG则不会使用RAG,而是调用数据计算相关的工具,甚至如果LLM本身具备一定的数据运算能力话,则完全不需要调用外部工具,直接输出答案。当然我们也可以将RAG和其他外部工具结合起来,协同解决更复杂的问题,如上图所示,在这个过程中,AI智能体会将任务拆解后,在每个步骤中分别调用不同的工具,或是RAG组件来输出最终答案。接下来我么就一起看下如何利用OpenVINOTM和LlamaIndex工具来构建一个Agentic-RAG系统。
第一步:模型转换与量化
LLM和Embedding模型是RAG系统中必要的组件,这里我们可以通过Optimum-intel CLI分别把他们转化为OpenVINO的IR格式,并进行量化压缩。
- 安装方法:
pip install optimum[openvino]
- LLM:
optimum-cli export openvino --model {llm_model_id} --task text-generation-with-past --trust-remote-code --weight-format int4 {llm_model_path}
- Embedding:
optimum-cli export openvino --model {embedding_model_id} --task feature-extraction {embedding_model_path}
第二步:模型任务初始化
目前基于OpenVINO的LLM,Embedding以及Reranker任务均已被集成在LlamaIndex框架中,开发者可以非常方便地利用导出的LLM和Embedding模型,将这两类任务在LlamaIndex中进行初始化。
- 安装方法:
pip install llama-index llama-index-llms-openvino llama-index-embeddings-openvino
- LLM:
from llama_index.llms.openvino import OpenVINOLLM
llm = OpenVINOLLM(
model_name=str(llm_model_path),
tokenizer_name=str(llm_model_path),
context_window=3900,
max_new_tokens=1000,
model_kwargs={"ov_config": ov_config},
device_map=llm_device.value,
completion_to_prompt=completion_to_prompt,
)
- Embedding:
from llama_index.embeddings.huggingface_openvino import OpenVINOEmbedding
embedding = OpenVINOEmbedding(folder_name=embedding_model_path, device=embedding_device.value)
第三步:构建RAG工具
接下来我们可以利用初始化后的LLM以及Embedding组件来构建RAG工具。第一步需要在LlamaIndex创建一个标准的RAG检索引擎,为了方便演示,该检索器仅使用默认的向量相似度搜索方式进行上下文过滤,如果想了解更完整的RAG搭建方法,可以参考OpenVINO notebooks仓库中的另一个示例:
https://github.com/openvinotoolkit/openvino_notebooks/tree/latest/notebooks/llm-rag-llamaindex
from llama_index.readers.file import PyMuPDFReader
from llama_index.core import VectorStoreIndex, Settings
from llama_index.core.tools import FunctionTool
Settings.embed_model = embedding
Settings.llm = llm
loader = PyMuPDFReader()
documents = loader.load(file_path=text_example_en_path)
index = VectorStoreIndex.from_documents(documents)
query_engine = index.as_query_engine(similarity_top_k=2)
在完成RAG检索引擎创建后,可以直接调用LllamaIndex的接口将它包装为一个Agent的工具,如下所示,同时需要添加对该工具的描述,以便LLM判断在什么时候调用什么工具。
from llama_index.core.tools import QueryEngineTool
budget_tool = QueryEngineTool.from_defaults(
query_engine,
name="Xeon6",
description="A RAG engine with some basic facts about Intel Xeon 6 processors with E-cores",
)
此外,为了演示Agentic-RAG对于复杂任务的拆解与多工具间的路由能力,我们还可以再准备两个单独的数学运算工具,供LLM选择。
def multiply(a: float, b: float) -> float:
"""Multiply two numbers and returns the product"""
return a * b
multiply_tool = FunctionTool.from_defaults(fn=multiply)
def add(a: float, b: float) -> float:
"""Add two numbers and returns the sum"""
return a + b
add_tool = FunctionTool.from_defaults(fn=add)
第四步:构建Agent任务流水线
因为该示例中用到的Llama3还不支持Function-call,所以这里我们可以创建了一个基于ReAct的Agent。在LlamaIndex中搭建Agent流水线只需要一行代码,通过ReActAgent.from_tools接口可以创建一个基础的ReAct Agent,并将刚才定义好的工具及LLM组件绑定到该Agent中。
agent = ReActAgent.from_tools([multiply_tool, add_tool, budget_tool], llm=llm, verbose=True)
接下来可以测试下效果,我们向Agent咨询了关于“4颗第六代Xeon CPU最大线程数“的问题,可以看到Agent首先会调用Xeon 6的RAG系统查询单颗CPU支持的最大线程数,然后再调用数学运算工具将获得的线程数乘以4,最后将得到的数字反馈给用户。
response = agent.chat("What's the maximum number of cores in an Intel Xeon 6 processor server with 4 sockets ? Go step by step, using a tool to do any math.")
Thought: The current language of the user is English. I need to use a tool to help me answer the question.
Action: Xeon6
Action Input: {'input': 'maximum cores in a single socket'}
Observation:
According to the provided context information, the maximum cores in a single socket is 144.
Thought: The current language of the user is English. I need to use a tool to help me answer the question.
Action: multiply
Action Input: {'a': 144, 'b': 4}
Observation: 576
Thought: The current language of the user is English. I can answer without using any more tools. I'll use the user's language to answer
Answer: The maximum number of cores in an Intel Xeon 6 processor server with 4 sockets is 576.
总结和展望
通过将Agent和RAG进行结合,我们直接提升LLM在解决复杂任务时的能力,相较于传统的RAG,Agentic-RAG更具产业落地价值。同时随着多智能体方法的引入,基于Agent的RAG将逐步取代传统RAG系统,实现更灵活,更精确的大语言模型应用业务体系。
参考资料:
- OpenVINO notebook:https://github.com/openvinotoolkit/openvino_notebooks/blob/latest/notebooks/llm-rag-llamaindex/llm-rag-llamaindex.ipynb
- OpenVINO LLM in LlamaIndex:https://docs.llamaindex.ai/en/stable/examples/llm/openvino/
- OpenVINO Embedding in LlamaIndex:
https://docs.llamaindex.ai/en/stable/examples/embeddings/openvino/
- OpenVINO Reranker in LlamaIndex:https://docs.llamaindex.ai/en/stable/examples/node_postprocessor/openvino_rerank/
更多推荐
所有评论(0)